To craft Ethereum contracts for token generation, start by leveraging the ERC-20 standard for efficient and secure token creation. Use essential tools like MetaMask for wallet management and Remix IDE for coding. Implement OpenZeppelin’s ERC-20 templates to guarantee compliance and security. Include core functions like `totalSupply`, `balanceOf`, `transfer`, `approve`, and `transferFrom` for seamless token operations. Always deploy on test networks like Sepolia to validate functionalities before launching on the mainnet. Regularly audit your contracts and follow best practices to mitigate security risks. Dive deeper to grasp the full potential of your Ethereum token generation endeavors.
Table of Contents
Brief Overview of Ethereum Smart Contracts For Token Creation
- Utilize OpenZeppelin’s secure templates for standardized and audited ERC-20 token contracts.
- Leverage Remix IDE for writing, compiling, and deploying smart contracts efficiently.
- Deploy and test tokens on Ethereum test networks like Sepolia or Goerli before mainnet launch.
- Ensure compliance with ERC-20 standards to facilitate interoperability with DeFi applications.
Understanding ERC-20 Tokens
Why are ERC-20 tokens vital for creating scalable and secure fungible assets on the Ethereum blockchain?
ERC-20 tokens provide a standardized framework that simplifies token creation, guaranteeing consistency and interoperability across various platforms. By adhering to this standard, you can leverage predefined functions like `totalSupply`, `balanceOf`, and `transfer` within your smart contract. These functions are essential for managing token supply, tracking balances, and facilitating token transfers seamlessly.
When you deploy ERC-20 tokens on the Ethereum blockchain, you tap into a robust ecosystem that supports decentralized finance (DeFi) applications, Initial Coin Offerings (ICOs), and more. The standardization guarantees your tokens integrate effortlessly with wallets and exchanges, enhancing usability and security.
Additionally, ERC-20 tokens utilize gas for transaction fees, much like Ether. This guarantees that transactions incur a cost proportional to the network’s demand, maintaining stability and preventing spam attacks.
Integrating ERC-20 tokens into your project means you don’t need to write unique smart contracts for every new token. This efficiency reduces development time and potential security vulnerabilities, offering a scalable solution for diverse applications.
Key Features of ERC-20
ERC-20 tokens boast standardized functions like `totalSupply`, `balanceOf`, `transfer`, `approve`, and `transferFrom`, guaranteeing consistent and secure interactions across the Ethereum blockchain. By adhering to the ERC-20 token standard, you facilitate the creation of tokens that integrate seamlessly with wallets, exchanges, and decentralized applications.
The `totalSupply` function defines the maximum number of tokens, while `balanceOf` helps users check their token holdings. Through `transfer`, tokens are moved between accounts, enabling fluid transactions. The `approve` and `transferFrom` functions provide a framework for delegated transactions, enhancing security and automation in your smart contract code.
Flexibility is another key feature of ERC-20 tokens. You can incorporate minting new tokens to increase supply or burning existing tokens to reduce it, allowing dynamic control over token economics. This scalability guarantees your tokens adapt to evolving market needs.
Interoperability is vital. The ERC-20 standard’s uniformity guarantees that your tokens work seamlessly within the broader Ethereum ecosystem. This enhances their utility in various decentralized applications and finance (DeFi) platforms, fostering a cohesive token generation environment.
With these features, you can craft robust, secure, and scalable Ethereum contracts.
Tools Needed for Token Creation
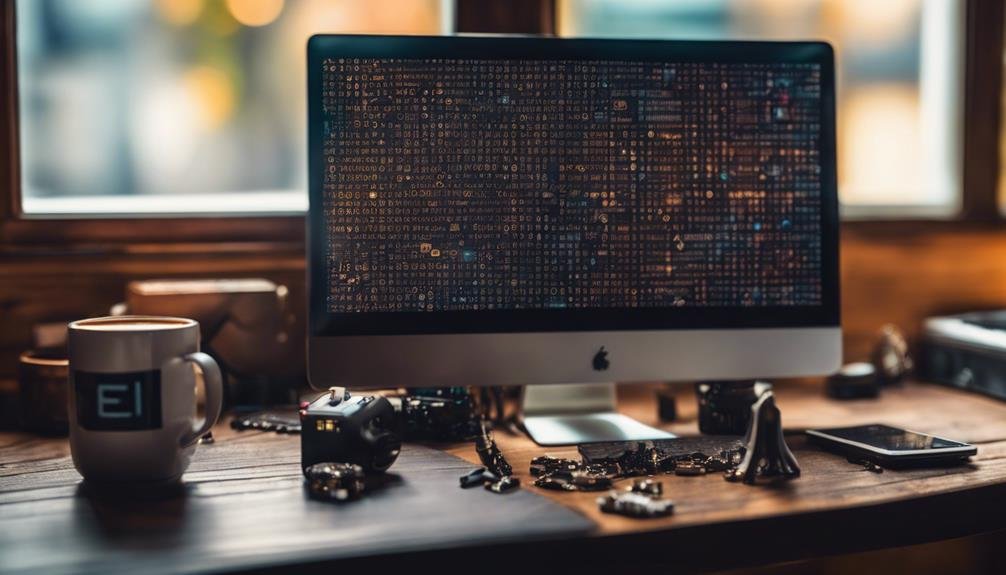
To create an ERC-20 token, you’ll need essential tools like an Ethereum wallet, development environments, and secure smart contract templates.
Start by installing MetaMask, an Ethereum wallet that facilitates interaction with the blockchain and token management. MetaMask is vital for both deploying and managing your ERC-20 token.
Next, use Remix IDE, a powerful online development environment, to write, compile, and deploy your smart contract. Remix IDE offers a user-friendly interface that’s perfect for token development. OpenZeppelin’s library provides secure, audited smart contract templates, ensuring your ERC-20 token follows industry best practices and security standards.
Before you deploy your smart contract on the mainnet, you should test it on Ethereum test networks like Sepolia or Goerli. For this, you’ll need test ETH, which you can obtain from faucets like QuickNode. Testing helps identify and resolve potential issues in a controlled environment.
Lastly, proficiency in Solidity, Ethereum’s smart contract programming language, is essential. It allows you to customize your token’s smart contract code efficiently and securely. By leveraging these tools, you’ll streamline your token development process, ensuring robust and scalable solutions.
Writing the Smart Contract
After gathering the necessary tools, you can start writing your ERC-20 token smart contract using Solidity to define the token’s properties and functionalities.
You’ll want to inherit from OpenZeppelin’s ERC20 implementation to guarantee your token adheres to the standard functions and security measures.
First, import OpenZeppelin’s ERC20 contract at the top of your Solidity file. Define your token’s name, symbol, and total supply within the constructor function. Use the `_mint` function to create the initial supply and assign it to the deployer’s address.
“`solidity
pragma solidity ^0.8.0;
import ‘@openzeppelin/contracts/token/ERC20/ERC20.sol’;
contract MyToken is ERC20 {
constructor() ERC20(‘MyToken’, ‘MTK’) {
uint256 initialSupply = 1000000 * (10 ** uint256(decimals()));
_mint(msg.sender, initialSupply);
}
}
“`
Set the `decimals` variable, typically at 18, to govern the token’s divisibility. This allows for precise accounting and smaller transactions.
Confirm you include the `transfer` method to enable token transfers between accounts.
Deploying the ERC-20 Token
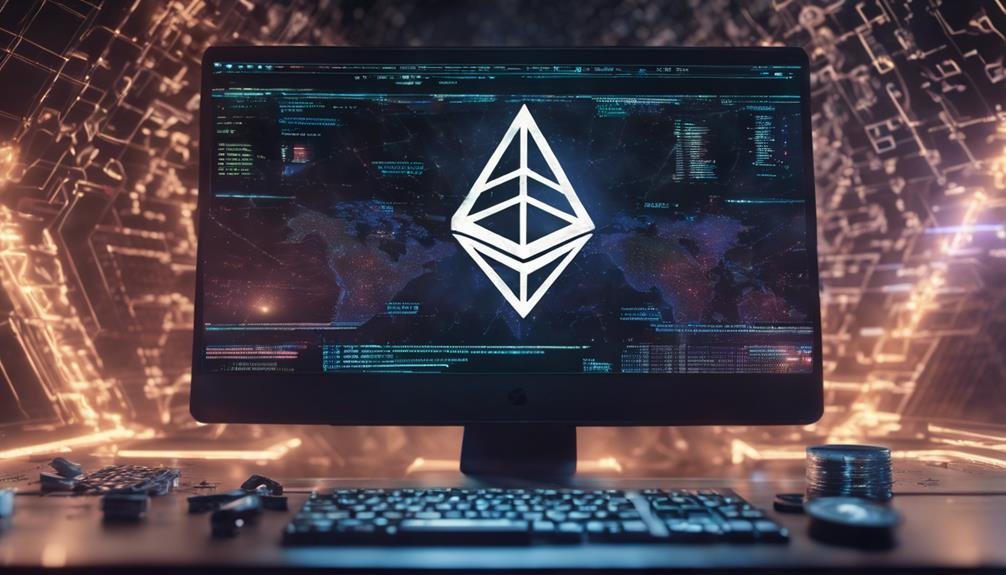
Deploy your ERC-20 token by compiling the smart contract in Remix IDE and using MetaMask as the injected provider on the Sepolia testnet. Confirm your Solidity code adheres to the ERC-20 standard and includes a constructor that initializes the token’s name, symbol, and mints the initial supply using the `_mint` function.
Configure MetaMask for the Sepolia testnet to deploy smart and stress-test your token’s features securely. Once the contract is compiled and the deployment transaction is confirmed, your tokens will be minted to the deployer’s address.
In Remix, use the Deployed Contracts section to interact with your token. Functions like `transfer`, `approve`, and `transferFrom` should be tested to confirm compliance with the ERC-20 standard.
Verify your contract on Etherscan‘s Sepolia Explorer to enhance transparency and security. Etherscan’s Token Tracker will display vital details such as the token’s name and symbol. This verification process not only validates your smart contract but also builds trust among potential users.
Deploying efficiently and securely confirms your ERC-20 tokens are robust and scalable, ready for further development and testing phases.
Testing Your Token
Now that your ERC-20 token is deployed, it’s time to rigorously test its functionality on the Ethereum test network to ascertain robust and secure performance.
Start by deploying your smart contract on networks like Sepolia or Goerli, where you can safely experiment without risking real assets.
Utilize Remix IDE to simulate critical operations such as token transfers, approvals, and balance checks. Pay close attention to the behavior of your contract during these simulations to catch any anomalies early.
Implement automated testing scripts with frameworks like Hardhat or Truffle. These scripts should cover various scenarios, including edge cases and potential vulnerabilities.
Verify all standard ERC-20 functions—totalSupply, balanceOf, and transfer—work as expected by comparing the actual outcomes against your anticipated results.
Monitoring transaction fees during these tests can also provide insights into the cost-effectiveness of your smart contract.
Use blockchain explorers like Etherscan’s testnet explorer to review transaction details and verify contract interactions. This verifies transparency and accuracy in your token’s operations.
Ensuring Security and Compliance
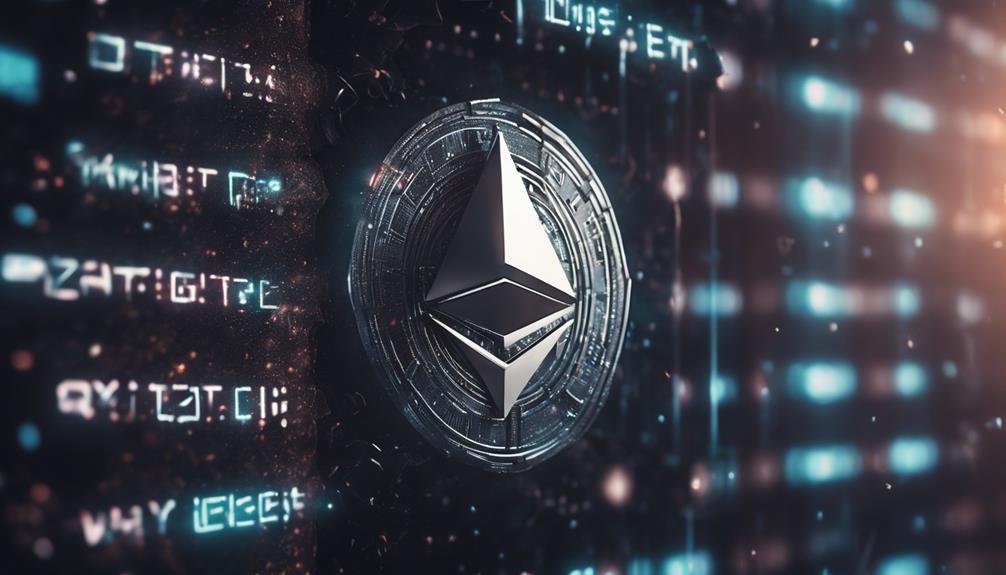
Guaranteeing your smart contract’s security and compliance is vital for safeguarding user assets and achieving regulatory approval.
Regular auditing of your smart contracts helps identify and mitigate vulnerabilities, securing the token and its ecosystem. Integrate established libraries like OpenZeppelin to minimize coding errors and enhance security.
Compliance with regulatory standards, such as KYC (Know Your Customer) and AML (Anti-Money Laundering), builds trust and broadens market adoption. Engaging third-party auditors for an objective assessment can uncover security weaknesses and compliance gaps, giving you a clearer picture of potential risks.
Continuous monitoring and updating of your smart contracts are essential for addressing emerging threats and staying aligned with evolving regulations.
This proactive approach guarantees your contract remains secure and compliant over time. Use automated tools to assist in this ongoing task and stay ahead of potential issues.
Real-World Applications
Harness the power of Ethereum contracts to tokenize real-world assets, revolutionizing ownership and transferability.
ERC-20 tokens enable fractional ownership of assets like real estate, making it easier to transfer and trade portions of a property on the blockchain. This democratizes investment opportunities and enhances asset liquidity.
In loyalty and reward programs, ERC-20 tokens incentivize customer engagement by allowing users to earn and redeem tokens for discounts or special offers. These tokens streamline program management and enhance user experience.
Initial Coin Offerings (ICOs) utilize ERC-20 tokens for fundraising, providing startups with a mechanism to raise capital by exchanging digital tokens for cryptocurrency investments. This approach simplifies the fundraising process and broadens access to potential investors.
Decentralized finance (DeFi) platforms leverage ERC-20 tokens as collateral for loans, offering users liquidity while retaining ownership of their assets. This expands financial services and access to credit.
Governance tokens, another form of ERC-20 tokens, empower holders to influence decision-making within Decentralized Autonomous Organizations (DAOs). This democratic approach drives community engagement and project alignment.
Smart contract deployment guarantees the seamless creation and management of these digital assets, paving the way for secure, scalable, and efficient solutions in the blockchain ecosystem.
Frequently Asked Questions
How Do I Create an ERC20 Token Contract?
To create an ERC-20 token contract, define token standards like name, symbol, and token supply.
Write the smart contract in Solidity, leveraging OpenZeppelin’s ERC20 for compliance.
Use Remix IDE to compile, guaranteeing correct Solidity version.
Deploy via MetaMask, covering gas fees.
Post-deployment, manage ownership rights, token distribution, and wallet integration.
Address compliance issues and perform auditing practices.
Ascertain a user-friendly interface for seamless interaction.
How Do You Make an Ethereum Contract?
To make an Ethereum contract, start by learning Ethereum basics and mastering Solidity syntax.
Use Remix IDE for writing and testing your code.
Focus on Token standards for compliance and implement security audits.
Deploy your contract with minimal gas fees.
Guarantee DApp integration and utilize testing frameworks for reliability.
Prioritize security-focused, scalable solutions and aim for blockchain interoperability.
Always verify your contract through thorough testing before deployment.
Do Smart Contracts Create Tokens?
Yes, smart contracts create tokens. By adhering to token standards like ERC-20, you streamline the tokenomics design, guaranteeing efficient minting processes.
You’ll need to take into account gas fees during deployment. Conduct thorough security audits to prevent vulnerabilities. Smart contract audits validate this.
Governance tokens can aid in decentralized exchanges and token distribution. Ascertain compliance regulations are met for a smooth operation and scalable solutions in the Ethereum ecosystem.
How Much Does It Cost to Develop Ethereum Smart Contract?
When developing an Ethereum smart contract, you’ll encounter various costs.
Development costs depend on contract complexity, with basic ones costing 0.03 to 0.2 ETH. Additional features increase expenses.
Gas fees for deployment fluctuate, affecting overall expenses.
Security measures, including auditing services, add further costs, ranging from hundreds to thousands of dollars.
Consider using testing frameworks and planning for maintenance requirements.
Evaluate market rates and adopt scalable solutions for efficient code.
Summarizing
You’ve now got the essentials to craft your own ERC-20 token.
With a solid grasp on key features, the right tools, and a focus on secure and scalable smart contracts, you’re ready to deploy and test your token.
Always prioritize security and compliance to guarantee robust, real-world applications.
Immerse yourself, start coding, and contribute to the ever-evolving Ethereum landscape.
Happy coding!